1. Overview
HealthBase is an application that evolved from a brown-field project, AddressBook, which consisted of 10KLOC. Over the course of 3 months, my team has gradually morphed the AddressBook into HealthBase by fortnightly meeting milestones that we set in the infancy stages of the project. |
This page encompasses my contributions to HealthBase. This includes the implementation of features, refactoring and documentation.
HealthBase is a desktop application designed to aid in the management of a hospital. It provides patient data input and management capabilities through a command-line interface for input and a graphical user interface for data display. Its main features (among others) include:
-
Patient registration into the HealthBase system
-
Patient dietary restriction(s) data entry and retrieval
-
Patient medication data entry and retrieval
-
Patient medical history data entry and retrieval
-
Patient visitor history data entry and retrieval
-
Real-time management of the number of visitors any given patient has
-
Patient appointment management for nurses
-
Patient discharge from the hospital
-
Patient check in for subsequent visits
2. Summary of contributions
-
Major enhancement: Added
addappt
command-
What it does: adds an appointment for a given patient. Each appointment includes the following information: type of procedure, procedure name, date and time of appointment and the Doctor-in-charge
-
Justification: this command provides a core functionality of the HealthBase (appointment data entry and retrieval).
-
Highlights: This feature currently allows for nurses to add and view upcoming appointments for patients. In V2.0 of HealtBase, doctors will be able to view the appointments they have with different patients.
-
-
Minor enhancement: Incorporate
appt
with theview
command -
Code contributed:
-
Other contributions:
-
Project management:
-
Bug Tester
-
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
3. Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Add appointment for patient: addappt
Add a scheduled appointment for a patient.
Format: addappt ic/NRIC type/TYPE pn/PROCEDURE_NAME dt/DD-MM-YYYY HH:MM doc/DOCTOR-IN-CHARGE
The only valid types are PROP (PROPAEDEUTIC), DIAG (DIAGNOSTIC), THP (THERAPEUTIC), SRG (SURGICAL).
Other inputs are not allowed.
|
It is useful to note that HealthBase does not permit appointments of duplicate date and time for any one patient. This is to prevent appointment clashes. |
The HealthBase System assumes that there are 31 days for all months. For the inputs of the dates 31 and 29 (for February), it is the onus of the user to ensure that
the input month has 31 days (for months except February) and 29 days for February in a leap year.
|
Example(s):
-
addappt ic/S1234567A type/SRG pn/Heart Bypass dt/27-04-2019 10:30 doc/Dr. Pepper
-
Type the example command into the input box as shown in the figure below and hit the Enter key:

-
The resulting output will be as shown in the figure below:

Register a new patient to the system: register
Register a new patient together with necessary information into the system.
Format: register ic/NRIC n/NAME p/PHONE_NUMBER e/EMAIL a/ADDRESS da/DRUG_ALLERGIES
If the patient is already registered, the command will not be allowed. |
The prefix da/ must be separated from the last input by a whitespace
|
Example(s):
-
register ic/S1234567A n/John Doe p/98765432 e/johnd@example.com a/311, Clementi Ave 2, #02-25 da/aspirin da/insulin
-
Type the example command into the input box as shown in the figure below and hit the Enter key:

-
The resulting output will be as shown in the figure below:

4. Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Addappt
Current implementation
The addappt
command provides functionality for users to add an appointment for a given patient.
This is done by adding appointment-related information to a given person, represented by a Person
object.
This allows for users to track the upcoming appointments for every patient.
The adding of appointment-related information is facilitated by the following classes:
-
AppointmentsList
, a list of-
Appointment
, each of which have a-
Type
-
-
A more detailed description of the classes follows below:
-
AppointmentsList
-
Every
Person
has anAppointmentsList
, the purpose of which is to store allAppointment
s belonging to aPerson
. -
A wrapper class around the internal representation of a list of appointments that exposes only a few select methods in the
List
API.-
The methods relevant to the
addappt
command from the API are:add
.
-
-
-
Appointment
-
Class encapsulating all the information about a particular appointment.
-
These information include the type of appointment (enum Type), name of the procedure, date and time of the appointment and the name of the doctor-in-charge of the appointment
-
-
-
Type
-
An enumeration that covers all the different types of medical procedures. The four types are:
-
PROPAEDEUTIC
, withPROP
as abbreviation -
DIAGNOSTIC
, withDIAG
as abbreviation -
THERAPEUTIC
, withTHP
as abbreviation -
SURGICAL
, withSRG
as abbreviation
-
-
Given below is an example usage scenario and how the relevant classes behave at each step.
Activity and sequence diagrams are different ways to represent the execution of a command: The activity diagram provides a general view of the execution and The sequence diagram provides an intricate view of the execution Both diagrams have been included to better aid you through the execution of the addappt command.
|
The user executes addappt ic/S1234567A type/SRG pn/Heart Bypass dt/27-04-2019 10:30 doc/Dr. Pepper
.
This command has the following intent: Record the following appointment to a patient with NRIC = S1234567A:
Appt. type | Procedure name | Date and time | Doctor-in-charge |
---|---|---|---|
|
Heart Bypass |
27-04-2019 10:30 |
Dr. Pepper |
The following activity diagram shows the execution of the addappt
command:
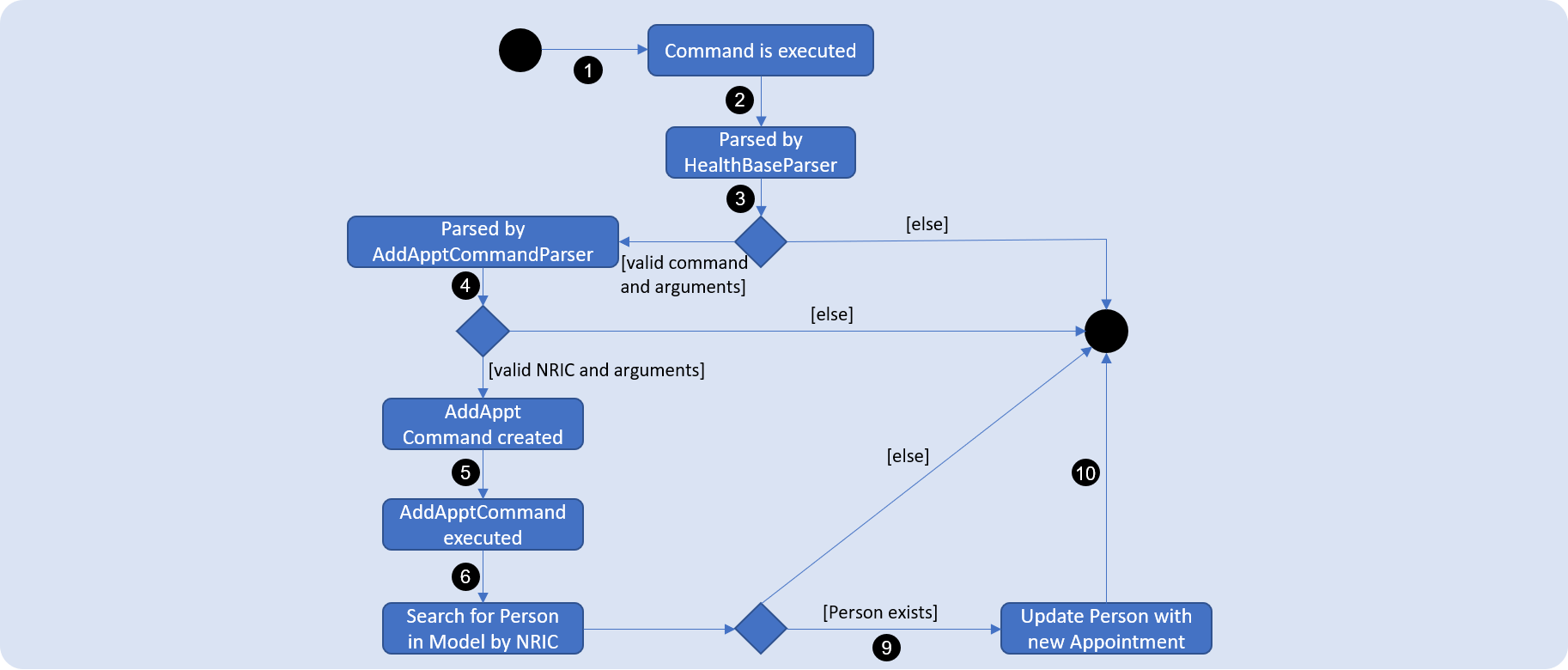
addappt
commandThe following sequence diagram shows the execution of the addappt
command:
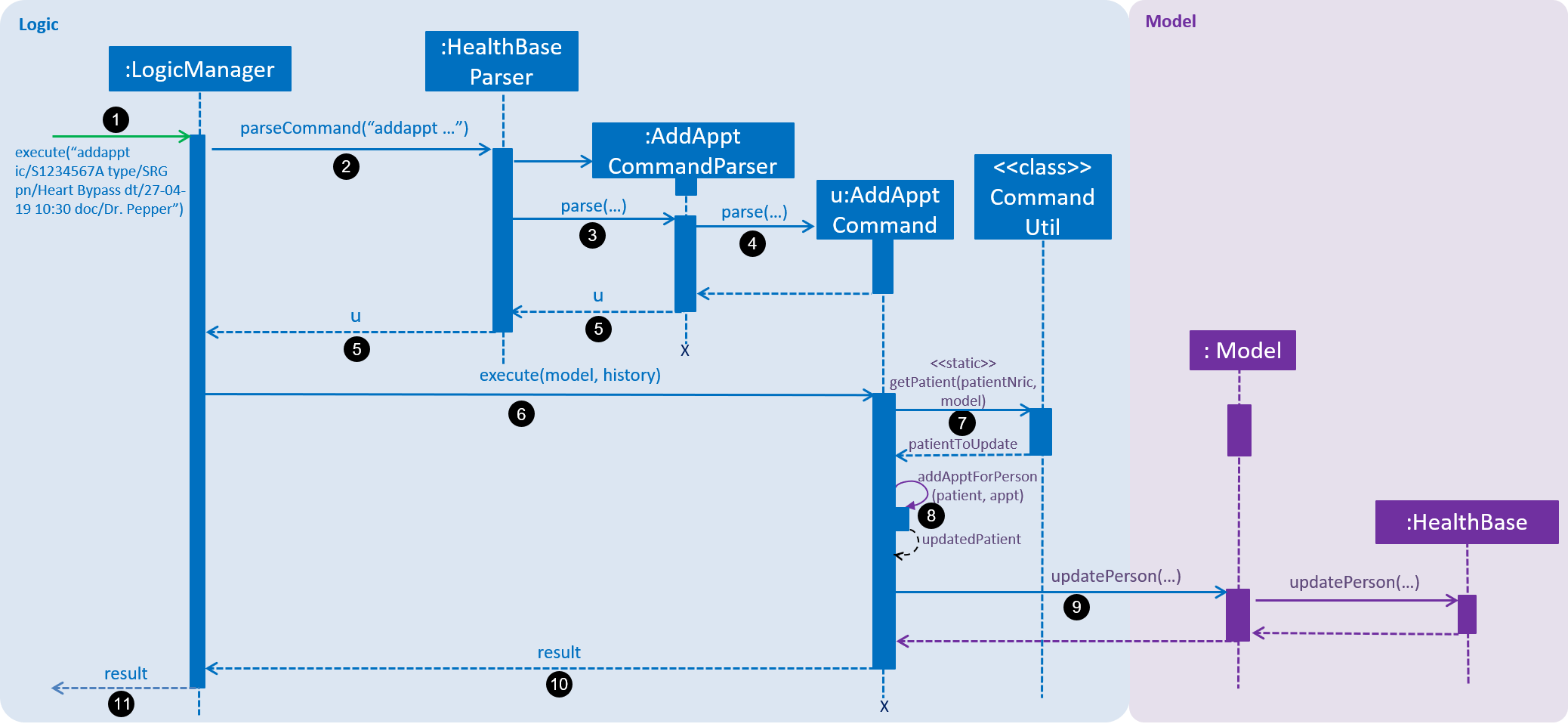
addappt
commandWith reference to the above figures, the following steps occur upon the execution of the addappt
command
(code snippets have been included for the first 4 steps to further aid understanding):
... @Override public CommandResult execute(String commandText) throws CommandException, ParseException { (1) logger.info("----------------[USER COMMAND][" + commandText + "]"); try { Command command = healthBaseParser.parseCommand(commandText); (2) return command.execute(model, history); } finally { history.add(commandText); } } ...
... public Command parseCommand(String userInput) throws ParseException { (2) final Matcher matcher = BASIC_COMMAND_FORMAT.matcher(userInput.trim()); if (!matcher.matches()) { throw new ParseException(String.format(MESSAGE_INVALID_COMMAND_FORMAT, HelpCommand.MESSAGE_USAGE)); } final String commandWord = matcher.group("commandWord"); final String arguments = matcher.group("arguments"); switch (commandWord) { case RegisterCommand.COMMAND_WORD: return new RegisterCommandParser().parse(arguments); ... case AddApptCommand.COMMAND_WORD: return new AddApptCommandParser().parse(arguments); (3) ... } ...
... @Override public AddApptCommand parse(String args) throws ParseException { (3) ArgumentMultimap argMultimap = ArgumentTokenizer.tokenize(args, PREFIX_NRIC, PREFIX_TYPE, PREFIX_PROCEDURE, PREFIX_DATE_TIME, PREFIX_DOCTOR); if (!arePrefixesPresent(argMultimap, PREFIX_NRIC, PREFIX_TYPE, PREFIX_PROCEDURE, PREFIX_DATE_TIME, PREFIX_DOCTOR) || !argMultimap.getPreamble().isEmpty()) { throw new ParseException(String.format(MESSAGE_INVALID_COMMAND_FORMAT, AddApptCommand.MESSAGE_USAGE)); } ... nric = new Nric(patientNric); appt = new Appointment(type, procedure, dateTime, doctor); return new AddApptCommand(nric, appt); (4) } ...
1 | The command is executed and passed to an instance of the LogicManager class, |
2 | which in turn executes HealthBaseParser::parseCommand . |
3 | The HealthBaseParser first parses the command word (addappt ), |
4 | then executes AddApptCommandParser::parse . |
5 | The AddApptCommandParser::parse method returns an AddApptCommand object which encapsulates the necessary information to update the Person 's Appointment (s).
Control is also passed back to the LogicManager . |
6 | Next, the instance of LogicManager calls AddApptCommand::execute . The AddApptCommand::execute method constructs a new Person object using all the details of the old Person with one exception: the
AppointmentsList is a copy of the original Person 's AppointmentsList with an added Appointment . |
7 | This is done by obtaining a Person object from the static method CommandUtil::getPatient |
8 | then adding the Appointment to the obtained Person . |
9 | This updated Person object is used to update the existing Person by using Model::updatePerson of the backing model. |
10 | Finally, the AddApptCommand::execute method terminates, returning a CommandResult with a success message. |
11 | The LogicManager returns the same CommandResult as the value of the LogicManager::execute method. |
12 | The command execution ends. |
If no/multiple patient(s) with that NRIC exist(s), then the AddApptCommand::execute method will throw a CommandException with the appropriate error message and the usage case will end.
|
For the sake of simplicity, some methods in AddApptCommand::execute are excluded from the diagram. These methods check for
the validity of the command and are not crucial in the sequence of events.
|
Design considerations
Aspect: Representation of types of medical procedures
-
Alternative 1 (Current implementation): Use an
Enum
forType
-
Pros: Makes for easier handling of incorrect values.
-
Cons: Requires more effort to filter and retrieve the different types.
-
-
Alternative 2: Have a switch case to handle the different types
-
Pros: Makes the process easier to handle.
-
Cons: Makes the code more difficult to read.
-
5. PROJECT: Accuride SG
An Android offline MRT app where you can get the fastest duration and the least transfers duration from one station to another.
Other features include:
-
Last train timings
-
Link to LTA’s Twitter page for updates on breakdowns/early closures (requires the Internet)